Please provide me with some context or a question so I can assist you. I need more information to understand what you’re looking for.
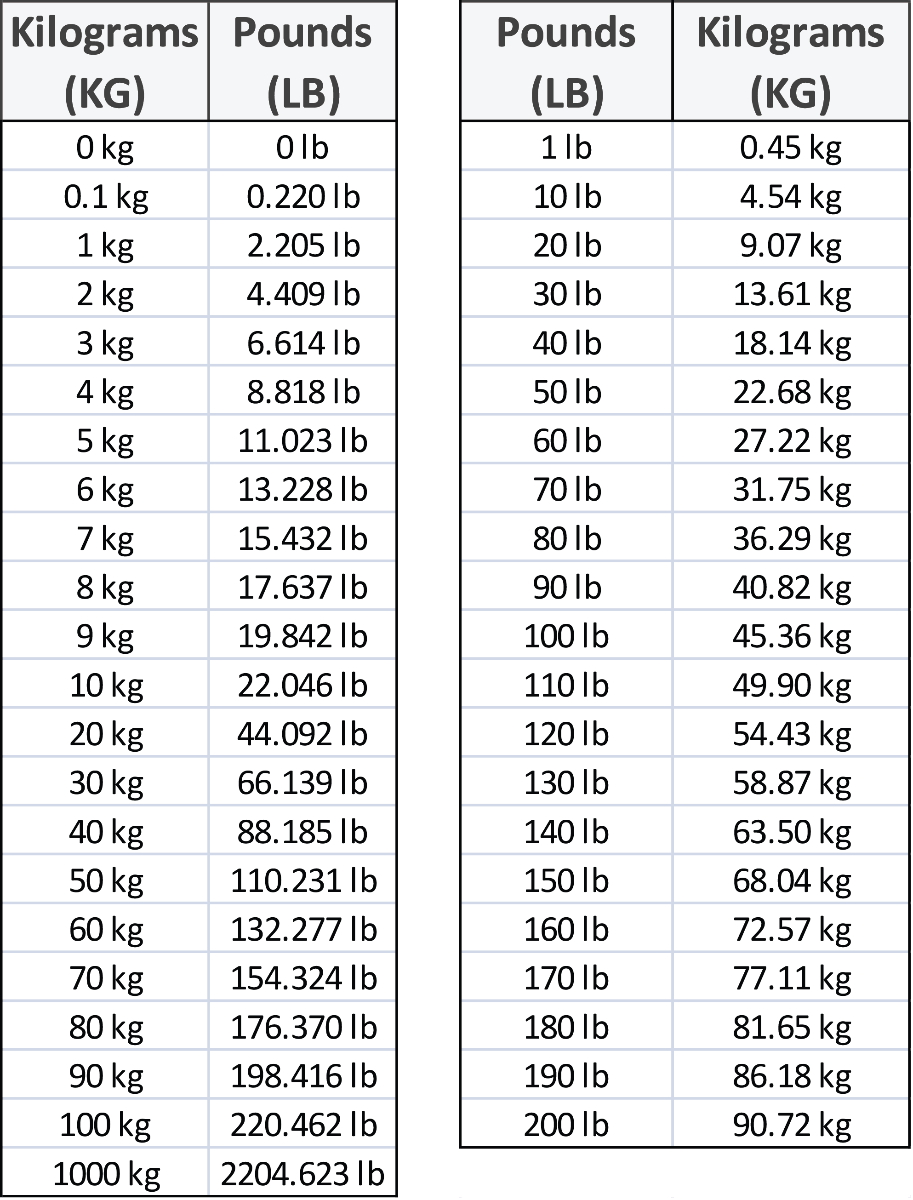
Image: bastalucid.weebly.com
For example, you could ask me:
- “What is the capital of France?”
- “Write a poem about the ocean.”
- “Can you summarize the plot of the movie ‘The Shawshank Redemption’?”
Let me know how I can help!
Please tell me what you need help with! I can provide information, generate creative content, translate languages, and answer your questions.
For example, you could ask me:
- “What is the best way to learn a new language?”
- “Write a short story about a time traveler.”
- “Translate ‘Hello world’ into Spanish.”
I’m eager to assist you! 😄
I need help understanding the concept of recursion in programming. Can you explain it in simple terms, perhaps with an example? I’d also appreciate it if you could provide some real-world applications of recursion.
Imagine you have a stack of boxes, and you want to count how many boxes are there. You could do it by taking one box off the top, counting it, and then repeating the process with the remaining stack. That’s essentially recursion!
Recursion in Programming
Recursion is a technique where a function calls itself within its own definition. Think of it like a set of Russian nesting dolls: each doll contains a smaller version of itself.
Here’s how it works:
- Base Case: This is the stopping condition. It defines when the recursion should end. In the box example, it would be when you reach an empty stack.
- Recursive Case: This is the part where the function calls itself, but with a smaller version of the problem. In our box example, it’s the step of taking a box off the top and counting it.
Example: Factorial
Let’s say you want to calculate the factorial of a number (e.g., 5! = 5 4 3 2 1). Here’s a recursive function to do it:
def factorial(n):
if n == 0: # Base case: Factorial of 0 is 1
return 1
else: # Recursive case: n! = n * (n-1)!
return n * factorial(n-1)
print(factorial(5)) # Output: 120
- Base case: When the input
n
is 0, the function returns 1. - Recursive Case: When
n
is greater than 0, the function multipliesn
by the result of calling itself withn-1
. This keeps going until it reaches the base case.
Real-World Applications
Recursion has many applications in programming, including:
- File System Navigation: Exploring directories and subdirectories can be done recursively.
- Tree Data Structures: Manipulating and traversing tree-like data structures (think of file system hierarchies) often uses recursion.
- Fractals: Generating complex geometric patterns like fractals relies on recursive principles.
- Sorting Algorithms: Algorithms like merge sort and quicksort use recursion to break down the problem into smaller parts.
- Artificial Intelligence: Recursion is fundamental in AI algorithms for tasks like natural language processing and game playing.
Key Points to Remember:
- Base Case: A recursive function must have a base case to prevent infinite recursion (like an endless loop).
- Stack Overflow: If the recursive function calls itself too many times, it can cause a stack overflow error. This is because each function call takes up space on the call stack, and it can eventually run out of space.
Recursion can be a powerful tool, but it’s important to understand how it works to use it effectively.

Image: www.wikihow.com
225kg To Lbs