Have you ever wondered how your favorite game stores its levels and character data, or how your favorite music streaming service plays songs on demand? The answer lies in a fundamental concept known as binary file manipulation. These files, composed of raw data, offer an efficient way to store various forms of information, from images and audio to complex 3D models. In this comprehensive guide, we’ll delve into the fascinating world of binary file operations, focusing on how Python and C++, two popular programming languages, can be used to read, write, and manipulate binary data effectively. We’ll also explore how vectors, a powerful data structure, can streamline this process, making it faster and more robust.
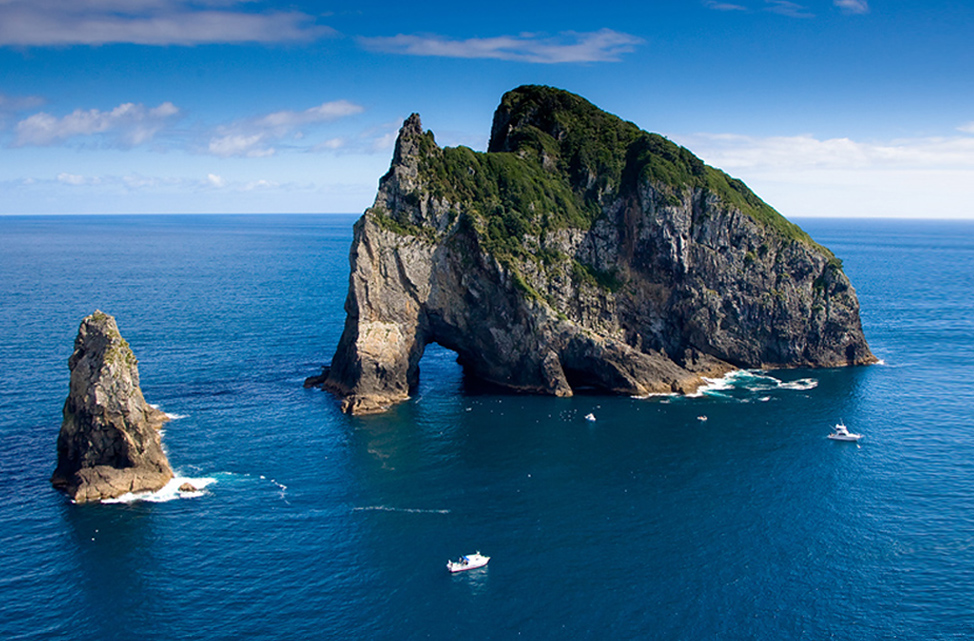
Image: nuclearrambo.com
Understanding binary file processing is crucial for developers working on diverse applications, from game development and multimedia software to scientific simulations and data analysis. Whether you’re a novice programmer or a seasoned professional, this guide will provide you with a solid foundation in binary file handling, empowering you to unleash the full potential of your programming skills.
The Power of Binary Files
Binary files are the building blocks of digital information storage. Unlike text files, which use characters to represent data, binary files represent information in its raw, uninterpreted form. Each character in a binary file is represented by a sequence of 0s and 1s, offering a compact and efficient way to store large amounts of data. Let’s take a closer look at the advantages of using binary files:
Advantages of Binary Files
- Compact Storage: Binary files are incredibly efficient in storing data, as they eliminate the need for character-based representation, leading to smaller file sizes.
- Speed: Reading and writing to binary files is significantly faster compared to text files, as the computer doesn’t need to interpret characters. This is particularly advantageous for handling large datasets or real-time applications.
- Versatility: Binary files can store virtually any type of data, including images, audio, video, and even complex structures like databases and program executables.
Python: A User-Friendly Approach
Python, renowned for its ease of use and readability, offers a straightforward approach to binary file handling. Let’s explore Python’s core features for reading and writing binary files.
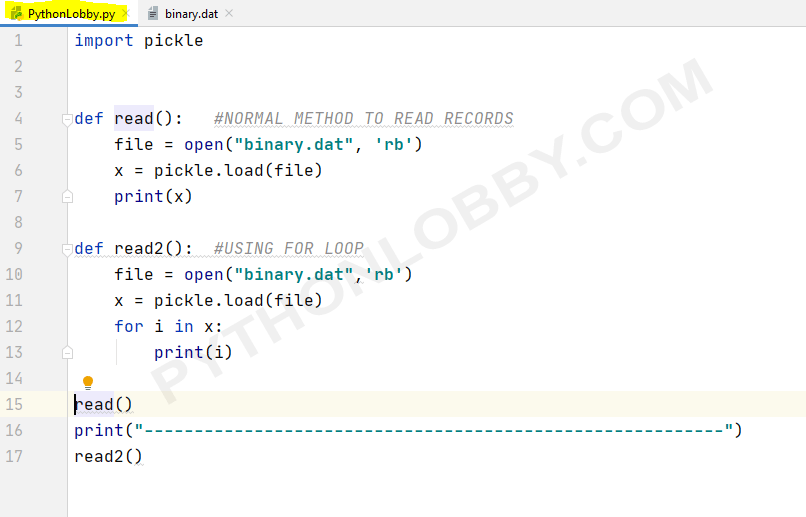
Image: pythonlobby.com
Reading Binary Files in Python
To read binary data in Python, the open()
function is utilized with the mode parameter set to 'rb'
, indicating read binary mode. The read()
method can then be used to read the entire file’s contents or a specific number of bytes.
# Example: Reading a binary image file
with open('image.jpg', 'rb') as file:
image_data = file.read()
print(image_data)
Writing Binary Files in Python
Writing binary data in Python is similarly achieved using the open()
function with the mode set to 'wb'
, signifying write binary mode. The write()
method is employed to write data into the file.
# Example: Writing a binary file with a list of integers
data = [1, 2, 3, 4, 5]
with open('data.bin', 'wb') as file:
file.write(bytes(data))
C++: Unleashing Speed and Control
C++, a powerful language known for its performance and control, provides a robust set of tools for binary file manipulation. Let’s dive into the fundamentals of reading and writing binary files in C++.
Reading Binary Files in C++
The cornerstone of binary file reading in C++ is the fstream
class. Using the ifstream
object for input, we can open binary files in read mode using the mode specifier ios::binary
. The read()
function then reads data from the file.
#include <fstream>
#include <iostream>
int main()
std::ifstream file("data.bin", std::ios::binary);
if (file.is_open())
int data;
while (file.read(reinterpret_cast<char*>(&data), sizeof(data)))
std::cout << data << " ";
file.close();
else
std::cerr << "Failed to open file." << std::endl;
return 0;
Writing Binary Files in C++
Similar to reading, the ofstream
object from the fstream
class is used for writing binary data. We open the file in write mode (ios::binary
) and employ the write()
function to write data to the file.
#include <fstream>
#include <iostream>
int main()
std::ofstream file("output.bin", std::ios::binary);
if (file.is_open())
int data[] = 10, 20, 30, 40, 50;
file.write(reinterpret_cast<char*>(data), sizeof(data));
file.close();
else
std::cerr << "Failed to open file." << std::endl;
return 0;
The Power of Vectors: Streamlining Binary Operations
Vectors, dynamic data structures that can store various data types, are a valuable tool for manipulating binary file data. They provide a flexible and efficient way to manage data before writing it to files or after reading it from files. Let’s explore how vectors can enhance binary file operations.
Reading Data into Vectors
When reading binary data from a file, vectors can be used to store the data in a structured and manageable way. In Python, we can directly read the data into a list, which is similar to a vector.
import struct
with open('data.bin', 'rb') as file:
data = file.read()
values = struct.unpack('<' + 'i' * int(len(data) / 4), data)
In C++, we can utilize the push_back()
method to add data elements to a vector as we read them from the file.
#include <fstream>
#include <vector>
#include <iostream>
int main()
std::ifstream file("data.bin", std::ios::binary);
std::vector<int> data;
if (file.is_open())
int value;
while (file.read(reinterpret_cast<char*>(&value), sizeof(value)))
data.push_back(value);
file.close();
else
std::cerr << "Failed to open file." << std::endl;
// Process the data in the vector
for (int num : data)
std::cout << num << " ";
std::cout << std::endl;
return 0;
Writing Data from Vectors
Vectors can also be used to efficiently write data to binary files. In Python, the struct
module enables packing data from a list into a binary string, which can then be written to a file.
import struct
data = [10, 20, 30, 40, 50]
packed_data = struct.pack('<' + 'i' * len(data), *data)
with open('output.bin', 'wb') as file:
file.write(packed_data)
In C++, the write()
function can be used to write the elements of a vector directly to a binary file.
#include <fstream>
#include <vector>
#include <iostream>
int main()
std::vector<int> data = 10, 20, 30, 40, 50;
std::ofstream file("output.bin", std::ios::binary);
if (file.is_open())
file.write(reinterpret_cast<char*>(data.data()), data.size() * sizeof(int));
file.close();
else
std::cerr << "Failed to open file." << std::endl;
return 0;
Real-world Applications
Binary file handling and vector manipulation are integral components in various real-world applications. Let’s explore a few examples:
1. Game Development
Imagine a complex 3D game level. It requires storing intricate data such as terrain details, object positions, and lighting information. This vast amount of data is efficiently represented in binary files, where vectors are used to organize and manipulate these data elements. During gameplay, the game engine reads binary files to load levels, textures, and other assets, delivering a seamless and interactive experience.
2. Image Processing
In image processing applications, images are often stored in binary file formats like JPEG or PNG. Binary file processing allows efficient access and manipulation of pixel data, enabling functions such as image resizing, color adjustment, and image filtering. Vectors can be used to represent and process individual pixels or groups of pixels for various image enhancement tasks.
3. Data Analysis
Data scientists and analysts often work with massive datasets stored in binary format for reasons of efficiency. Reading and manipulating these binary files using vectors can significantly improve the speed and efficiency of data processing and analysis, enabling better data insights and faster decision-making.
Read In Python C++ Write Binary File From Vector
https://youtube.com/watch?v=FKKo9Weo5Ew
Conclusion
Mastering binary file operations is a fundamental skill for any programmer. Python and C++, with their powerful libraries and data structures, offer a robust foundation for reading, writing, and manipulating binary data. Vectors, as dynamic and efficient data structures, further streamline these operations, enhancing performance and making data management more seamless. From game development to image processing and data analysis, binary file handling and vectors play a vital role in shaping the digital world around us. Armed with the knowledge gained from this guide, you are empowered to explore the intricacies of binary files and harness their potential to create innovative and powerful applications.
We encourage you to experiment with the provided examples, venture into more advanced binary file operations, and explore the vast possibilities that binary files offer. Share your experiences and creative implementations in the comments section, and let’s continue to unlock the full potential of this fundamental aspect of computer science!